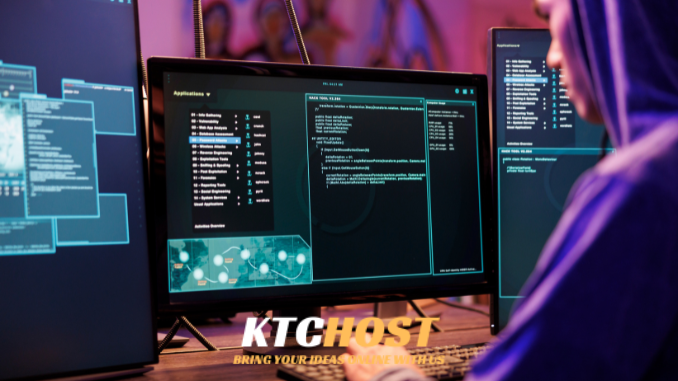
A Jenkinsfile is used to define the pipeline as code in Jenkins. It can be written in either Declarative or Scripted syntax. Below is an overview of both types with examples:
1. Declarative Pipeline Syntax
The Declarative Pipeline syntax is the simpler and more structured of the two. It is designed to make it easier to define your CI/CD pipeline in a clear and readable format.
Basic structure of a Declarative Pipeline:
pipeline {
agent any // This tells Jenkins to run the pipeline on any available agent
environment {
MY_ENV_VAR = 'SomeValue' // Define environment variables
}
stages {
stage('Build') {
steps {
script {
echo 'Building the project...'
// Commands to build your project
}
}
}
stage('Test') {
steps {
script {
echo 'Running tests...'
// Commands to run tests
}
}
}
stage('Deploy') {
steps {
script {
echo 'Deploying to production...'
// Commands to deploy your application
}
}
}
}
post {
success {
echo 'Build succeeded!'
}
failure {
echo 'Build failed.'
}
}
}
Key sections:
pipeline
: This block wraps the entire Jenkins pipeline.agent
: Defines the environment where the pipeline will run (e.g.,any
,docker
, or specific node labels).stages
: Contains different stages of your pipeline (e.g.,Build
,Test
,Deploy
).steps
: Specifies the individual tasks within each stage.post
: Contains actions to run after the pipeline finishes (e.g., success or failure notifications).
2. Scripted Pipeline Syntax
The Scripted Pipeline is more flexible and provides the ability to write more complex logic within the pipeline using Groovy scripts. It’s ideal when you need more control or advanced workflows.
Basic structure of a Scripted Pipeline:
node {
try {
stage('Build') {
echo 'Building the project...'
// Build commands go here
}
stage('Test') {
echo 'Running tests...'
// Test commands go here
}
stage('Deploy') {
echo 'Deploying to production...'
// Deployment commands go here
}
} catch (Exception e) {
currentBuild.result = 'FAILURE'
throw e
} finally {
echo 'Pipeline finished'
}
}
Key differences from Declarative:
- Scripted pipelines are written in Groovy and offer full flexibility, allowing for complex logic, loops, and more control over the flow.
- There is no structured
stages
block; stages are manually defined usingstage('StageName')
.
Example: Simple Jenkinsfile for a Node.js Project
Here’s an example of a Declarative Pipeline Jenkinsfile for a Node.js project that includes the stages for install, test, and deploy:
pipeline {
agent any
environment {
NODE_ENV = 'production'
}
stages {
stage('Install Dependencies') {
steps {
script {
echo 'Installing dependencies...'
sh 'npm install'
}
}
}
stage('Run Tests') {
steps {
script {
echo 'Running tests...'
sh 'npm test'
}
}
}
stage('Deploy') {
steps {
script {
echo 'Deploying to production...'
sh 'npm run deploy'
}
}
}
}
post {
success {
echo 'Build succeeded!'
}
failure {
echo 'Build failed.'
}
}
}
Key Jenkinsfile Concepts:
node
: Defines where the pipeline runs (can be on any agent or specific labels).sh
: Executes shell commands.environment
: Defines environment variables, which can be used across stages.post
: Defines actions that happen after the pipeline execution, like sending success/failure notifications.
Conclusion:
The Declarative pipeline is the preferred approach for most users due to its simplicity and readability. The Scripted pipeline offers more control and flexibility, ideal for complex workflows or when more customization is needed. You can choose based on your team’s needs, but in most cases, Declarative Pipelines are sufficient for typical CI/CD setups.
Would you like to see more advanced examples or details on integrating Jenkins with other tools? if YES then keep monitor the BLOGS for more examples in upcoming days