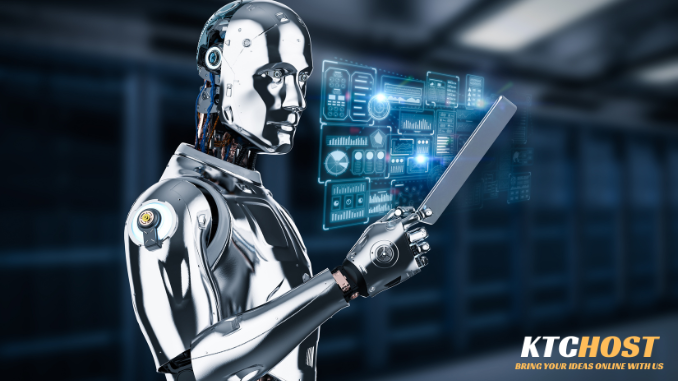
🚀 Deploy an Application Using Terraform and Ansible: A Beginner’s Guide
Combining Terraform and Ansible helps automate infrastructure deployment and configuration management efficiently. In this guide, we’ll:
✅ Use Terraform to provision an AWS EC2 instance
✅ Use Ansible to configure the server and deploy an application
Step 1: Install Terraform and Ansible
Install Terraform
For Linux/macOS:
wget https://releases.hashicorp.com/terraform/1.6.0/terraform_1.6.0_linux_amd64.zip
unzip terraform_1.6.0_linux_amd64.zip
sudo mv terraform /usr/local/bin/
terraform --version
For Windows:
- Download Terraform from Terraform Official Website
- Extract and add it to your system’s PATH
- Run
terraform --version
to verify
Install Ansible
For Ubuntu/Debian:
sudo apt update
sudo apt install ansible -y
For CentOS/RHEL:
sudo yum install epel-release -y
sudo yum install ansible -y
For macOS:
brew install ansible
Step 2: Configure AWS Credentials
Ensure you have AWS CLI installed and configured:
aws configure
Enter your AWS Access Key, Secret Key, Region, and Output format.
Step 3: Write a Terraform Script to Create an EC2 Instance
Create a new directory for your project:
mkdir terraform-ansible-demo && cd terraform-ansible-demo
Create a main.tf
file:
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe1f0" # Amazon Linux AMI (update as per your region)
instance_type = "t2.micro"
key_name = "my-key" # Ensure this key exists in AWS
security_groups = ["default"]
provisioner "local-exec" {
command = "echo ${self.public_ip} > inventory.ini"
}
tags = {
Name = "Terraform-Ansible-Instance"
}
}
Step 4: Initialize and Apply Terraform
Run the following commands:
terraform init
terraform apply -auto-approve
Once completed, Terraform will create an inventory.ini file with the instance’s public IP.
Step 5: Create an Ansible Inventory File
Modify inventory.ini
to look like this:
[web]
<EC2-PUBLIC-IP> ansible_user=ec2-user ansible_ssh_private_key_file=my-key.pem
Replace <EC2-PUBLIC-IP>
with the instance’s actual IP.
Step 6: Write an Ansible Playbook to Install and Deploy an Application
Create a file called deploy.yml
:
---
- name: Deploy Application
hosts: web
become: yes
tasks:
- name: Install Apache
yum:
name: httpd
state: present
- name: Start Apache Service
service:
name: httpd
state: started
enabled: yes
- name: Create a Sample Web Page
copy:
dest: /var/www/html/index.html
content: "<h1>Welcome to Ansible Automated Deployment</h1>"
- name: Restart Apache
service:
name: httpd
state: restarted
Step 7: Run the Ansible Playbook
Execute the playbook using:
ansible-playbook -i inventory.ini deploy.yml
Step 8: Verify the Deployment
Open a browser and visit:
http://<EC2-PUBLIC-IP>
You should see “Welcome to Ansible Automated Deployment”.
Step 9: Cleanup
When done, destroy the infrastructure:
terraform destroy -auto-approve
🎯 Summary of What We Did:
✅ Used Terraform to create an AWS EC2 instance
✅ Used Ansible to install and configure Apache
✅ Successfully deployed a sample web page
💡 Next Steps:
- Automate database setup
- Deploy a full-stack application
- Integrate CI/CD pipelines
Happy Automating! 🚀
#Terraform, #Ansible, #DevOps, #InfrastructureAutomation, #CloudComputing, #AWS, #Automation, #BeginnersGuide