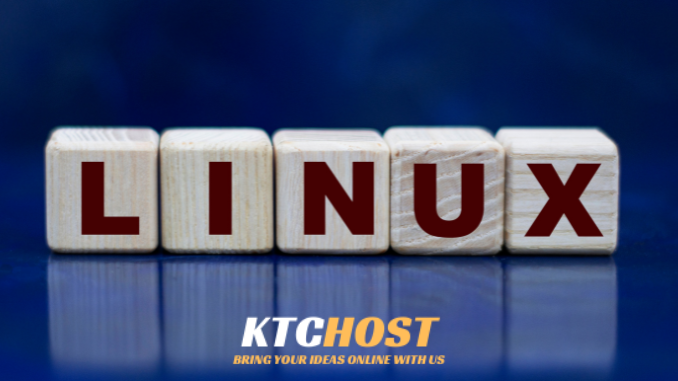
To execute commands on a Linux machine from a Jenkinsfile, you can use the sh
step, which allows you to run shell commands. This can be done in either a Declarative Pipeline or Scripted Pipeline.
Example: Simple Jenkinsfile to Run Linux Commands (Declarative Jenkinsfile)
pipeline {
agent any // This can be adjusted to a specific agent or label if needed
stages {
stage('Run Linux Commands') {
steps {
script {
// Running a simple shell command on Linux
sh 'echo "Hello, Jenkins!"'
sh 'ls -l' // List the directory contents
sh 'uptime' // Display the system uptime
}
}
}
}
post {
success {
echo 'Linux commands executed successfully.'
}
failure {
echo 'Error executing Linux commands.'
}
}
}
Key Points:
sh
Step: This is used to execute Linux shell commands. Anything you can run in the terminal, you can run through Jenkins.- For example,
sh 'echo "Hello, Jenkins!"'
will printHello, Jenkins!
in the console output.
- For example,
- Multiple Commands: You can execute multiple commands in a single
sh
step, or use separatesh
steps for different commands. - Error Handling: The
post
block helps handle success or failure scenarios after the pipeline completes.
Example: Simple Jenkinsfile to Run Linux Commands (Scripted Jenkinsfile)
node {
stage('Run Linux Commands') {
try {
// Running multiple shell commands
sh 'echo "Starting Linux Command Execution"'
sh 'df -h' // Display disk space usage
sh 'top -n 1' // Display system processes once
} catch (Exception e) {
currentBuild.result = 'FAILURE'
throw e
}
}
}
Notes:
- The
sh
step can run any shell commands (likels
,df
,top
, etc.) as if you were typing them directly into the terminal. - Ensure that the Jenkins agent has the necessary permissions and environment to execute the commands.
If you need to run specific commands that require elevated privileges, you might need to configure Jenkins or the agent with proper sudo permissions, which can be done by using sudo
in the command, but it’s important to configure it safely to avoid security risks.