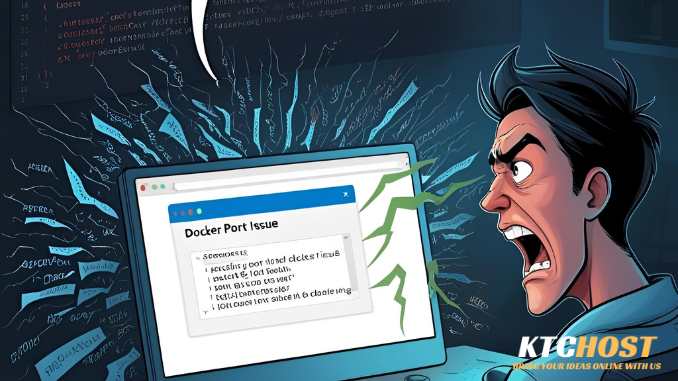
For instance, to run a simple Nginx web server, the command would be
docker run -d -p 80:80 nginx
This command starts an Nginx container, detaches it (-d
), and maps port 80 of the host to port 80 of the container. If port 80 on the host is already in use by another container or any other process, and you run:
docker run -d -p 80:80 nginx
Then Docker will throw an error similar to:
docker: Error response from daemon: driver failed programming external connectivity on endpoint <container_name> (β¦): Error starting userland proxy: listen tcp 0.0.0.0:80: bind: address already in use.
Why?
Because Docker uses host port binding, and two containers (or any processes) cannot listen on the same port on the host (in this case, port 80).
π§ Solutions:
β 1. Use a different host port:
If you still want to run another Nginx container, map it to a different port on the host:
docker run -d -p 8080:80 nginx
Now:
- Host
http://localhost:8080
β Containerport 80
β 2. Stop the container (or process) using port 80:
If the existing service on port 80 isn’t needed anymore:
docker ps # to find the container
docker stop <container_id>
Then re-run your original docker run
command.
β 3. Check what’s using port 80:
sudo lsof -i :80
# or
sudo netstat -tuln | grep :80
This tells you which process is occupying port 80.
Bash script that checks if a port is already in use and then starts a Docker container only if the port is free. If the port is in use, it will show an appropriate message.
β
Script: safe_docker_run.sh
#!/bin/bash
# --- CONFIGURABLE ---
HOST_PORT=80
CONTAINER_PORT=80
IMAGE_NAME="nginx"
CONTAINER_NAME="safe_nginx"
# --- CHECK IF PORT IS IN USE ---
if lsof -i :$HOST_PORT &>/dev/null; then
echo "β Port $HOST_PORT is already in use. Cannot start container."
echo "π Process using the port:"
lsof -i :$HOST_PORT
exit 1
else
echo "β
Port $HOST_PORT is free. Starting container..."
docker run -d --name $CONTAINER_NAME -p $HOST_PORT:$CONTAINER_PORT $IMAGE_NAME
echo "π Container '$CONTAINER_NAME' started and bound to port $HOST_PORT."
fi
π§ͺ How to Use:
- Save it as
safe_docker_run.sh
- Make it executable:
chmod +x safe_docker_run.sh
- Run it:
./safe_docker_run.sh