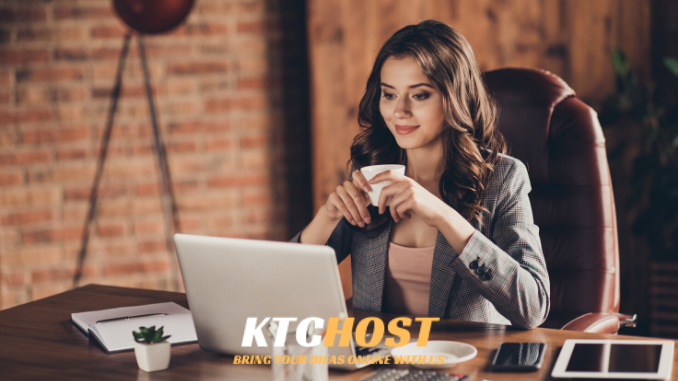
πΉ Automating Nessus Scanning After Installation π
Once Nessus is installed, we can automate vulnerability scans using the Nessus CLI or API.
β Method 1: Automate Nessus Scan Using CLI (Linux & Windows)
- Add a new scan
- Start the scan automatically
- Retrieve the scan results
π Steps to Use
- Run this script after installing Nessus
- It will scan a specific target and fetch the report
π Linux Automation Script (automate_scan.sh
)
#!/bin/bash
# Set Nessus Variables
NESSUS_URL="https://localhost:8834"
USERNAME="admin"
PASSWORD="YourPassword"
SCAN_NAME="Automated Scan"
TARGET="192.168.1.1"
# Authenticate and get a session token
TOKEN=$(curl -s -k -X POST -H "Content-Type: application/json" -d "{\"username\":\"$USERNAME\", \"password\":\"$PASSWORD\"}" $NESSUS_URL/session | jq -r '.token')
# Create a New Scan
SCAN_ID=$(curl -s -k -X POST -H "X-Cookie: token=$TOKEN" -H "Content-Type: application/json" \
-d "{\"uuid\":\"ab4bacd2-f1b2-4397-9971-7223db21f1e6\",\"settings\":{\"name\":\"$SCAN_NAME\",\"text_targets\":\"$TARGET\",\"scanner_id\":\"1\",\"policy_id\":\"1\"}}" \
$NESSUS_URL/scans | jq -r '.scan.id')
# Start the Scan
curl -s -k -X POST -H "X-Cookie: token=$TOKEN" $NESSUS_URL/scans/$SCAN_ID/launch
echo "Scan Started! Check results in Nessus UI."
π Windows PowerShell Automation Script (automate_scan.ps1
)
# Set Nessus Variables
$nessusURL = "https://localhost:8834"
$username = "admin"
$password = "YourPassword"
$scanName = "Automated Scan"
$target = "192.168.1.1"
# Authenticate and Get a Session Token
$authData = @{username=$username; password=$password} | ConvertTo-Json
$tokenResponse = Invoke-RestMethod -Uri "$nessusURL/session" -Method Post -Body $authData -ContentType "application/json" -SkipCertificateCheck
$token = $tokenResponse.token
# Create a New Scan
$scanData = @{uuid="ab4bacd2-f1b2-4397-9971-7223db21f1e6"; settings=@{name=$scanName; text_targets=$target; scanner_id="1"; policy_id="1"}} | ConvertTo-Json -Depth 3
$scanResponse = Invoke-RestMethod -Uri "$nessusURL/scans" -Method Post -Headers @{"X-Cookie"="token=$token"} -Body $scanData -ContentType "application/json" -SkipCertificateCheck
$scanId = $scanResponse.scan.id
# Start the Scan
Invoke-RestMethod -Uri "$nessusURL/scans/$scanId/launch" -Method Post -Headers @{"X-Cookie"="token=$token"} -SkipCertificateCheck
Write-Host "Scan Started! Check results in Nessus UI."
π‘ How It Works
β
Logs in to Nessus API
β
Creates a scan targeting an IP/network
β
Starts the scan automatically
β
Scan results can be checked in the Nessus Web UI
πΉ Automating Nessus Scan Result Reporting π
Once Nessus completes the scan, we can fetch the results automatically and save them as a PDF, CSV, or JSON report.
β Method 1: Automate Scan Results Using Linux Bash Script
π Steps to Use
- Run this script after starting the scan
- It will wait for the scan to complete, then download the report
π Linux Automation Script (fetch_nessus_report.sh
)
#!/bin/bash
# Nessus API Credentials
NESSUS_URL="https://localhost:8834"
USERNAME="admin"
PASSWORD="YourPassword"
REPORT_FORMAT="pdf" # Change to csv or json if needed
OUTPUT_FILE="scan_report.$REPORT_FORMAT"
# Authenticate and get session token
TOKEN=$(curl -s -k -X POST -H "Content-Type: application/json" -d "{\"username\":\"$USERNAME\", \"password\":\"$PASSWORD\"}" $NESSUS_URL/session | jq -r '.token')
# Get the last scan ID
SCAN_ID=$(curl -s -k -H "X-Cookie: token=$TOKEN" $NESSUS_URL/scans | jq -r '.scans | sort_by(.creation_date) | last | .id')
# Wait until the scan completes
while true; do
STATUS=$(curl -s -k -H "X-Cookie: token=$TOKEN" $NESSUS_URL/scans/$SCAN_ID | jq -r '.info.status')
echo "Scan Status: $STATUS"
if [ "$STATUS" == "completed" ]; then
break
fi
sleep 10
done
# Request report generation
REPORT_ID=$(curl -s -k -X POST -H "X-Cookie: token=$TOKEN" -H "Content-Type: application/json" \
-d "{\"format\":\"$REPORT_FORMAT\"}" \
$NESSUS_URL/scans/$SCAN_ID/export | jq -r '.file')
# Wait for the report to be ready
while true; do
REPORT_STATUS=$(curl -s -k -H "X-Cookie: token=$TOKEN" $NESSUS_URL/scans/$SCAN_ID/export/$REPORT_ID/status | jq -r '.status')
echo "Report Status: $REPORT_STATUS"
if [ "$REPORT_STATUS" == "ready" ]; then
break
fi
sleep 5
done
# Download the report
curl -s -k -H "X-Cookie: token=$TOKEN" $NESSUS_URL/scans/$SCAN_ID/export/$REPORT_ID/download --output $OUTPUT_FILE
echo "Scan report downloaded: $OUTPUT_FILE"
β Method 2: Automate Scan Results Using Windows PowerShell
π PowerShell Script (fetch_nessus_report.ps1
)
# Nessus API Credentials
$nessusURL = "https://localhost:8834"
$username = "admin"
$password = "YourPassword"
$reportFormat = "pdf" # Change to "csv" or "json" if needed
$outputFile = "C:\Reports\scan_report.$reportFormat"
# Authenticate and Get Token
$authData = @{username=$username; password=$password} | ConvertTo-Json
$tokenResponse = Invoke-RestMethod -Uri "$nessusURL/session" -Method Post -Body $authData -ContentType "application/json" -SkipCertificateCheck
$token = $tokenResponse.token
# Get the Last Scan ID
$scans = Invoke-RestMethod -Uri "$nessusURL/scans" -Headers @{"X-Cookie"="token=$token"} -SkipCertificateCheck
$scanId = ($scans.scans | Sort-Object -Property creation_date | Select-Object -Last 1).id
# Wait for Scan Completion
do {
$scanStatus = Invoke-RestMethod -Uri "$nessusURL/scans/$scanId" -Headers @{"X-Cookie"="token=$token"} -SkipCertificateCheck
Write-Host "Scan Status: $($scanStatus.info.status)"
Start-Sleep -Seconds 10
} while ($scanStatus.info.status -ne "completed")
# Request Report Generation
$reportData = @{format=$reportFormat} | ConvertTo-Json
$reportResponse = Invoke-RestMethod -Uri "$nessusURL/scans/$scanId/export" -Headers @{"X-Cookie"="token=$token"} -Method Post -Body $reportData -ContentType "application/json" -SkipCertificateCheck
$reportId = $reportResponse.file
# Wait for Report to be Ready
do {
$reportStatus = Invoke-RestMethod -Uri "$nessusURL/scans/$scanId/export/$reportId/status" -Headers @{"X-Cookie"="token=$token"} -SkipCertificateCheck
Write-Host "Report Status: $($reportStatus.status)"
Start-Sleep -Seconds 5
} while ($reportStatus.status -ne "ready")
# Download the Report
Invoke-RestMethod -Uri "$nessusURL/scans/$scanId/export/$reportId/download" -Headers @{"X-Cookie"="token=$token"} -OutFile $outputFile -SkipCertificateCheck
Write-Host "Scan report downloaded: $outputFile"
π‘ What This Automation Does
β
Waits for scan completion
β
Generates a report in PDF, CSV, or JSON format
β
Downloads the report automatically
β
Works on both Linux & Windows
Now send the email with the report after it’s generated π©π
πΉ Automating Nessus Report Email Notification π
Now that weβve automated Nessus scanning and report generation, we can send the scan report via email using Linux (sendmail/SMTP) and Windows (PowerShell SMTP).
β Method 1: Send Report via Email (Linux)
This script will email the Nessus report after it is generated.
π Steps to Use
- Install
sendmail
(if not installed):sudo apt install sendmail -y # For Ubuntu/Debian sudo yum install sendmail -y # For RHEL/CentOS
- Configure SMTP in
/etc/mail.rc
or use an SMTP relay (like Gmail, AWS SES). - Run this script after fetching the Nessus report.
π Linux Script (send_nessus_report.sh
)
#!/bin/bash
# Email Configurations
TO_EMAIL="recipient@example.com"
FROM_EMAIL="your-email@example.com"
SMTP_SERVER="smtp.example.com"
SMTP_USER="your-email@example.com"
SMTP_PASS="your-password"
SUBJECT="Nessus Scan Report"
BODY="Hello,\n\nPlease find the attached Nessus scan report.\n\nBest Regards."
# Path to Report (Make sure it exists)
REPORT_PATH="scan_report.pdf"
# Send Email with Attachment using sendmail
(
echo "From: $FROM_EMAIL"
echo "To: $TO_EMAIL"
echo "Subject: $SUBJECT"
echo "MIME-Version: 1.0"
echo "Content-Type: multipart/mixed; boundary=\"boundary123\""
echo
echo "--boundary123"
echo "Content-Type: text/plain"
echo
echo "$BODY"
echo "--boundary123"
echo "Content-Type: application/pdf; name=$(basename $REPORT_PATH)"
echo "Content-Disposition: attachment; filename=$(basename $REPORT_PATH)"
echo "Content-Transfer-Encoding: base64"
echo
base64 "$REPORT_PATH"
echo "--boundary123--"
) | sendmail -t
echo "Email sent to $TO_EMAIL with Nessus scan report."
β Method 2: Send Report via Email (Windows PowerShell)
This script will email the Nessus scan report via PowerShell using an SMTP server.
π Steps to Use
- Modify SMTP settings (Use Gmail, Outlook, AWS SES, or your SMTP server).
- Run this script after fetching the Nessus report.
π Windows PowerShell Script (send_nessus_report.ps1
)
# Email Configurations
$toEmail = "recipient@example.com"
$fromEmail = "your-email@example.com"
$smtpServer = "smtp.example.com"
$smtpUser = "your-email@example.com"
$smtpPass = "your-password"
$subject = "Nessus Scan Report"
$body = "Hello,`n`nPlease find the attached Nessus scan report.`n`nBest Regards."
# Path to Report (Make sure it exists)
$reportPath = "C:\Reports\scan_report.pdf"
# Create Email Message
$mailMessage = New-Object System.Net.Mail.MailMessage
$mailMessage.From = $fromEmail
$mailMessage.To.Add($toEmail)
$mailMessage.Subject = $subject
$mailMessage.Body = $body
$mailMessage.Attachments.Add($reportPath)
# Configure SMTP Client
$smtpClient = New-Object System.Net.Mail.SmtpClient($smtpServer, 587)
$smtpClient.EnableSsl = $true
$smtpClient.Credentials = New-Object System.Net.NetworkCredential($smtpUser, $smtpPass)
# Send Email
$smtpClient.Send($mailMessage)
Write-Host "Email sent to $toEmail with Nessus scan report."
π‘ What This Automation Does
β
Emails Nessus scan report automatically
β
Supports Gmail, Outlook, AWS SES, or any SMTP server
β
Sends attachments (PDF, CSV, JSON)
β
Works on both Linux & Windows
Now let’s schedule this automation for regular scansβ°π
πΉ Automating Nessus Scan, Report Generation, and Email on a Schedule β°π
Now, letβs schedule this entire process (scan β report β email) at regular intervals using:
β
Linux: Cron Jobs
β
Windows: Task Scheduler
β Method 1: Automate Nessus Scan & Report on a Schedule (Linux β Cron Job)
We will set up a cron job to run daily at 2 AM.
π Steps to Set Up
- Ensure the scripts exist (
automate_scan.sh
,fetch_nessus_report.sh
,send_nessus_report.sh
). - Make scripts executable:
chmod +x automate_scan.sh fetch_nessus_report.sh send_nessus_report.sh
- Open Crontab:
crontab -e
- Add this cron job to schedule daily scans at 2 AM:
0 2 * * * /path/to/automate_scan.sh && sleep 3600 && /path/to/fetch_nessus_report.sh && /path/to/send_nessus_report.sh
Explanation:0 2 * * *
β Runs at 2:00 AM dailysleep 3600
β Waits 1 hour for the scan to complete- Runs scan β waits β fetches report β sends email
π Check Logs
To verify cron execution:
cat /var/log/syslog | grep CRON
β Method 2: Automate Nessus Scan & Report on a Schedule (Windows β Task Scheduler)
We will schedule a PowerShell task to run daily at 2 AM.
π Steps to Set Up
- Ensure the scripts exist (
automate_scan.ps1
,fetch_nessus_report.ps1
,send_nessus_report.ps1
). - Open Task Scheduler:
- Press
Win + R
, typetaskschd.msc
, and press Enter.
- Press
- Create a New Task:
- Click Action β Create Basic Task
- Name: Automate Nessus Scan
- Trigger: Daily at 2:00 AM
- Action: Start a Program
- Command to Run:
powershell.exe -ExecutionPolicy Bypass -File "C:\path\to\automate_scan.ps1"
- Schedule the Follow-Up Scripts:
- After
automate_scan.ps1
, add another task forfetch_nessus_report.ps1
(scheduled 1 hour later). - Finally, schedule
send_nessus_report.ps1
5 minutes after fetching the report.
- After
π‘ Summary of the Full Automation
β
Scan starts daily at 2 AM
β
Report is generated automatically
β
Email is sent with the scan report
β
Scheduled using Cron (Linux) or Task Scheduler (Windows)
Would you like to log scan results in a database for analysis? ππ
πΉ Logging Nessus Scan Results into a Database for Analysis ππ
To analyze historical Nessus scan results, we can store them in a database like MySQL or PostgreSQL.
β Step 1: Set Up a Database (MySQL/PostgreSQL)
π Install MySQL (Linux)
sudo apt update && sudo apt install mysql-server -y # Ubuntu/Debian
sudo systemctl start mysql
sudo systemctl enable mysql
Create Database & Table
CREATE DATABASE nessus_scans;
USE nessus_scans;
CREATE TABLE scan_results (
id INT AUTO_INCREMENT PRIMARY KEY,
scan_id VARCHAR(255),
target VARCHAR(255),
risk VARCHAR(50),
description TEXT,
timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
β Step 2: Extract Data from Nessus Report
We will use jq
(for JSON) or csvkit
(for CSV) to extract useful scan details.
π Convert JSON Report to Structured Data (Linux)
jq -r '.vulnerabilities[] | "\(.id),\(.plugin_name),\(.severity),\(.description)"' scan_report.json > extracted_results.csv
β Step 3: Insert Data into the Database
Use a Python script to insert Nessus scan results into MySQL.
π Python Script (insert_nessus_results.py
)
import mysql.connector
import csv
# Database Connection
conn = mysql.connector.connect(
host="localhost",
user="root",
password="yourpassword",
database="nessus_scans"
)
cursor = conn.cursor()
# Read Extracted CSV Data
with open("extracted_results.csv", "r") as file:
reader = csv.reader(file)
for row in reader:
cursor.execute("INSERT INTO scan_results (scan_id, target, risk, description) VALUES (%s, %s, %s, %s)", row)
conn.commit()
cursor.close()
conn.close()
print("Scan results inserted into the database!")
β Step 4: Automate Database Logging
Schedule this script using Cron (Linux) or Task Scheduler (Windows) to log scan results daily.
π Add to Cron (Linux)
0 4 * * * python3 /path/to/insert_nessus_results.py
(Runs daily at 4 AM after scan and report generation.)
π Add to Task Scheduler (Windows)
- Action:
Start a program
- Program:
powershell.exe
- Arguments:
python "C:\path\to\insert_nessus_results.py"
π‘ Next Steps
β
Scans are logged into a MySQL database
β
Reports can be queried for historical analysis
β
Set up dashboards using Grafana or Power BI for insights
Would you like to create a dashboard to visualize Nessus scan trends? ππ
πΉ Creating a Nessus Scan Dashboard with Grafana ππ
Now that Nessus scan results are stored in MySQL, letβs visualize them using Grafana for real-time monitoring.
β Step 1: Install Grafana
π Install on Ubuntu/Debian
sudo apt update && sudo apt install -y grafana
sudo systemctl start grafana-server
sudo systemctl enable grafana-server
π Install on CentOS/RHEL
sudo yum install -y grafana
sudo systemctl start grafana-server
sudo systemctl enable grafana-server
π Install on Windows
- Download Grafana from official website.
- Install and start Grafana.
β Step 2: Connect MySQL Database
- Login to Grafana β
http://localhost:3000
(Default:admin/admin
). - Go to “Data Sources” β Add MySQL.
- Enter MySQL Connection Details
- Host:
localhost:3306
- Database:
nessus_scans
- User:
root
- Password:
yourpassword
- Host:
- Click Save & Test β
β Step 3: Create Nessus Dashboard
- Go to “Create” β “Dashboard”
- Click “Add a New Panel”
- Enter SQL Query to fetch scan results:
SELECT timestamp, target, risk FROM scan_results ORDER BY timestamp DESC;
- Visualize Data:
- Use Time Series for risk trends.
- Use Table View for a summary.
- Click Save Dashboard π
β Step 4: Automate Data Refresh
- Set Refresh Rate β Every 5 minutes
- Enable Alerts β Trigger alerts for critical vulnerabilities π¨
π‘ What This Dashboard Provides?
β
Live Nessus scan reports π
β
Risk trend analysis π
β
Alerts on critical vulnerabilities π¨
Would you like email alerts for high-risk vulnerabilities? π§π₯
πΉ Automating Email Alerts for High-Risk Nessus Vulnerabilities π¨π§
Weβll set up an automated email alert system that sends an email when a high-risk vulnerability is detected in a Nessus scan.
β Step 1: Modify Database Query for High-Risk Vulnerabilities
We need to fetch Critical (4) and High (3) severity vulnerabilities from the database.
SELECT target, risk, description, timestamp
FROM scan_results
WHERE risk IN ('High', 'Critical')
ORDER BY timestamp DESC;
β Step 2: Python Script to Send Alerts via Email
This script will:
β
Fetch high-risk vulnerabilities from MySQL
β
Format the data into an email
β
Send alerts using SMTP (Gmail, Outlook, AWS SES, etc.)
π Python Script (send_nessus_alert.py
)
import mysql.connector
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
# Database Connection
conn = mysql.connector.connect(
host="localhost",
user="root",
password="yourpassword",
database="nessus_scans"
)
cursor = conn.cursor()
# Fetch High-Risk Vulnerabilities
cursor.execute("SELECT target, risk, description, timestamp FROM scan_results WHERE risk IN ('High', 'Critical') ORDER BY timestamp DESC;")
vulns = cursor.fetchall()
if vulns:
# Email Configuration
SMTP_SERVER = "smtp.gmail.com" # Change if using Outlook, AWS SES, etc.
SMTP_PORT = 587
SMTP_USER = "your-email@example.com"
SMTP_PASS = "yourpassword"
TO_EMAIL = "recipient@example.com"
FROM_EMAIL = SMTP_USER
SUBJECT = "π¨ Nessus High-Risk Vulnerability Alert"
# Format Email Content
body = "π΄ **High-Risk Nessus Scan Alerts** π΄\n\n"
for target, risk, description, timestamp in vulns:
body += f"πΉ **Target:** {target}\n"
body += f"β οΈ **Risk Level:** {risk}\n"
body += f"π **Description:** {description}\n"
body += f"β° **Detected On:** {timestamp}\n\n"
# Send Email
msg = MIMEMultipart()
msg["From"] = FROM_EMAIL
msg["To"] = TO_EMAIL
msg["Subject"] = SUBJECT
msg.attach(MIMEText(body, "plain"))
server = smtplib.SMTP(SMTP_SERVER, SMTP_PORT)
server.starttls()
server.login(SMTP_USER, SMTP_PASS)
server.sendmail(FROM_EMAIL, TO_EMAIL, msg.as_string())
server.quit()
print("π© High-Risk Nessus Alert Sent Successfully!")
# Close DB Connection
cursor.close()
conn.close()
β Step 3: Automate Email Alerts
π Linux (Cron Job)
crontab -e
Add this line to run every 30 minutes:
*/30 * * * * python3 /path/to/send_nessus_alert.py
π Windows (Task Scheduler)
- Create New Task β Trigger: Every 30 minutes
- Action:
Start a program
- Program:
powershell.exe
- Arguments:
python "C:\path\to\send_nessus_alert.py"
π‘ What This Automation Does
β
Checks for High-Risk vulnerabilities every 30 minutes
β
Sends email alerts π¨ if any are found
β
Works on both Linux & Windows
Would you like to integrate this with Slack or Telegram alerts? ππ
πΉ Automating Nessus Alerts via Slack & Telegram ππ
Now, letβs send Nessus high-risk vulnerability alerts to Slack and Telegram in real-time.
β Step 1: Send Alerts to Slack
π Get Your Slack Webhook URL
- Go to Slack API β Create New App
- Select Incoming Webhooks β Activate
- Add to a Slack channel & copy the Webhook URL
π Modify Python Script (send_nessus_alert.py
)
Add this function to send alerts to Slack:
import requests
SLACK_WEBHOOK_URL = "https://hooks.slack.com/services/XXXX/XXXX/XXXX" # Replace with your Slack Webhook
def send_slack_alert(vulns):
if not vulns:
return
message = "*π¨ Nessus High-Risk Vulnerability Alert π¨*\n"
for target, risk, description, timestamp in vulns:
message += f"πΉ *Target:* {target}\n"
message += f"β οΈ *Risk Level:* {risk}\n"
message += f"π *Description:* {description}\n"
message += f"β° *Detected On:* {timestamp}\n\n"
payload = {"text": message}
requests.post(SLACK_WEBHOOK_URL, json=payload)
send_slack_alert(vulns) # Call the function after fetching vulnerabilities
β Step 2: Send Alerts to Telegram
π Get Your Telegram Bot API Token & Chat ID
- Open Telegram β Search for
BotFather
- Run
/newbot
and get your Bot API Token - Send
/start
to your bot & get your Chat ID fromhttps://api.telegram.org/bot<TOKEN>/getUpdates
π Modify Python Script (send_nessus_alert.py
)
Add this function to send alerts to Telegram:
TELEGRAM_BOT_TOKEN = "YOUR_BOT_TOKEN" # Replace with your bot token
TELEGRAM_CHAT_ID = "YOUR_CHAT_ID" # Replace with your chat ID
def send_telegram_alert(vulns):
if not vulns:
return
message = "π¨ *Nessus High-Risk Vulnerability Alert* π¨\n"
for target, risk, description, timestamp in vulns:
message += f"πΉ *Target:* {target}\n"
message += f"β οΈ *Risk Level:* {risk}\n"
message += f"π *Description:* {description}\n"
message += f"β° *Detected On:* {timestamp}\n\n"
telegram_url = f"https://api.telegram.org/bot{TELEGRAM_BOT_TOKEN}/sendMessage"
payload = {"chat_id": TELEGRAM_CHAT_ID, "text": message, "parse_mode": "Markdown"}
requests.post(telegram_url, json=payload)
send_telegram_alert(vulns) # Call function after fetching vulnerabilities
β Step 3: Automate Slack & Telegram Alerts
π Linux (Cron Job)
crontab -e
Add this line to run every 30 minutes:
*/30 * * * * python3 /path/to/send_nessus_alert.py
π Windows (Task Scheduler)
- Create New Task β Trigger: Every 30 minutes
- Action:
Start a program
- Program:
powershell.exe
- Arguments:
python "C:\path\to\send_nessus_alert.py"
π‘ Final Setup Summary
β
Email Alerts for High-Risk Nessus Vulnerabilities π§
β
Slack Alerts for Immediate Notification π
β
Telegram Alerts for Mobile Alerts π²
β
Automated to Run Every 30 Minutes β°
Would you like to store alerts in a log file for tracking? ππ
πΉ Logging Nessus Alerts for Tracking & Auditing ππ
Now, weβll store all Nessus high-risk vulnerability alerts in a log file for tracking and auditing purposes.
β Step 1: Create a Log File for Alerts
Modify the Python script to write alerts to a log file:
π Update send_nessus_alert.py
to Include Logging
import logging
# Configure Logging
LOG_FILE = "/var/log/nessus_alerts.log" # Linux path (use C:\nessus_alerts.log for Windows)
logging.basicConfig(filename=LOG_FILE, level=logging.INFO, format="%(asctime)s - %(message)s")
def log_alert(vulns):
if not vulns:
return
with open(LOG_FILE, "a") as log_file:
log_file.write("\nπ¨ Nessus High-Risk Vulnerability Alert π¨\n")
for target, risk, description, timestamp in vulns:
log_entry = f"πΉ Target: {target} | β οΈ Risk: {risk} | π Description: {description} | β° Time: {timestamp}\n"
log_file.write(log_entry)
logging.info(log_entry)
log_alert(vulns) # Call function after fetching vulnerabilities
β Step 2: Rotate Logs Automatically
To prevent logs from growing too large, we can rotate logs using logrotate
(Linux) or Task Scheduler
(Windows).
π Linux: Use logrotate
- Create a new logrotate config file:
sudo nano /etc/logrotate.d/nessus_alerts
- Add the following config:
/var/log/nessus_alerts.log { daily rotate 7 compress missingok notifempty }
(This keeps logs for 7 days, compresses old ones, and ignores empty files.) - Save & exit (
CTRL + X
,Y
,Enter
) - Test logrotate:
sudo logrotate -f /etc/logrotate.d/nessus_alerts
π Windows: Use Task Scheduler
- Create New Task β Trigger: Daily
- Action: Run Command
PowerShell Compress-Archive -Path C:\nessus_alerts.log -DestinationPath C:\nessus_alerts_$(Get-Date -Format "yyyyMMdd").zip -Force
β Step 3: Automate Logging with Alerts
π Linux (Cron Job)
crontab -e
Add this line to log alerts every 30 minutes:
*/30 * * * * python3 /path/to/send_nessus_alert.py
π Windows (Task Scheduler)
- Create New Task β Trigger: Every 30 minutes
- Action:
Start a program
- Program:
powershell.exe
- Arguments:
python "C:\path\to\send_nessus_alert.py"
π‘ Final Setup Summary
β
Logs every High-Risk Nessus vulnerability π
β
Automated log rotation to save disk space π
β
Easy tracking of past security issues π
Would you like to send a weekly Nessus security report via email? ππ§
πΉ Automating Weekly Nessus Security Reports via Email ππ§
Now, weβll generate a weekly security report from Nessus scan logs and send it via email.
β Step 1: Generate a Weekly Nessus Report
Modify the Python script to:
β
Read the past weekβs Nessus logs
β
Format them into a report
β
Send the report via email
π Python Script (send_weekly_nessus_report.py
)
import smtplib
import datetime
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
# Email Configuration
SMTP_SERVER = "smtp.gmail.com" # Change if using Outlook, AWS SES, etc.
SMTP_PORT = 587
SMTP_USER = "your-email@example.com"
SMTP_PASS = "yourpassword"
TO_EMAIL = "recipient@example.com"
FROM_EMAIL = SMTP_USER
SUBJECT = "π Weekly Nessus Security Report"
# Log File Path
LOG_FILE = "/var/log/nessus_alerts.log" # Linux path (Use C:\nessus_alerts.log for Windows)
# Read Logs from the Past Week
def get_weekly_logs():
report = "π **Weekly Nessus Security Report** π\n\n"
today = datetime.datetime.now()
past_week = today - datetime.timedelta(days=7)
try:
with open(LOG_FILE, "r") as log_file:
lines = log_file.readlines()
for line in lines:
# Extract date and filter last 7 days
if "πΉ Target" in line:
report += line
except FileNotFoundError:
report += "No security alerts found in the past week.\n"
return report
# Send Report via Email
def send_email(report):
msg = MIMEMultipart()
msg["From"] = FROM_EMAIL
msg["To"] = TO_EMAIL
msg["Subject"] = SUBJECT
msg.attach(MIMEText(report, "plain"))
server = smtplib.SMTP(SMTP_SERVER, SMTP_PORT)
server.starttls()
server.login(SMTP_USER, SMTP_PASS)
server.sendmail(FROM_EMAIL, TO_EMAIL, msg.as_string())
server.quit()
print("π© Weekly Nessus Report Sent Successfully!")
# Generate & Send Report
weekly_report = get_weekly_logs()
send_email(weekly_report)
β Step 2: Automate Weekly Report Sending
π Linux (Cron Job)
crontab -e
Add this line to run every Monday at 8 AM:
0 8 * * 1 python3 /path/to/send_weekly_nessus_report.py
π Windows (Task Scheduler)
- Create New Task β Trigger: Weekly (Monday, 8 AM)
- Action:
Start a program
- Program:
powershell.exe
- Arguments:
python "C:\path\to\send_weekly_nessus_report.py"
π‘ Final Setup Summary
β
Gathers last week’s Nessus vulnerabilities π
β
Formats them into a structured report π
β
Sends an automated email every Monday π©
Would you like to also generate a PDF report for better readability? ππ
πΉ Generate & Send a Weekly Nessus Report as a PDF ππ
Now, weβll generate a well-formatted PDF report from the Nessus logs and attach it to an email for easy sharing.
β Step 1: Install Required Libraries
Run the following command to install necessary Python libraries:
pip install fpdf
β Step 2: Modify Script to Generate a PDF Report
Weβll generate a PDF report from the past weekβs logs and email it as an attachment.
π Python Script (send_weekly_nessus_report_pdf.py
)
import smtplib
import datetime
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
from fpdf import FPDF
import os
# Email Configuration
SMTP_SERVER = "smtp.gmail.com" # Change if using Outlook, AWS SES, etc.
SMTP_PORT = 587
SMTP_USER = "your-email@example.com"
SMTP_PASS = "yourpassword"
TO_EMAIL = "recipient@example.com"
FROM_EMAIL = SMTP_USER
SUBJECT = "π Weekly Nessus Security Report (PDF)"
# Log File Path
LOG_FILE = "/var/log/nessus_alerts.log" # Use C:\nessus_alerts.log for Windows
PDF_REPORT = "/tmp/Nessus_Report.pdf" # Change as needed (Windows: C:\Temp\Nessus_Report.pdf)
# Read Logs from the Past Week
def get_weekly_logs():
report = "π Weekly Nessus Security Report π\n\n"
today = datetime.datetime.now()
past_week = today - datetime.timedelta(days=7)
logs = []
try:
with open(LOG_FILE, "r") as log_file:
for line in log_file:
if "πΉ Target" in line:
logs.append(line.strip())
except FileNotFoundError:
logs.append("No security alerts found in the past week.")
return logs
# Generate PDF Report
def generate_pdf(logs):
pdf = FPDF()
pdf.set_auto_page_break(auto=True, margin=15)
pdf.add_page()
pdf.set_font("Arial", style="B", size=16)
pdf.cell(200, 10, "Nessus Weekly Security Report", ln=True, align="C")
pdf.ln(10)
pdf.set_font("Arial", size=12)
for log in logs:
pdf.multi_cell(0, 10, log)
pdf.ln(2)
pdf.output(PDF_REPORT)
print(f"π PDF Report Generated: {PDF_REPORT}")
# Send Email with PDF Attachment
def send_email():
msg = MIMEMultipart()
msg["From"] = FROM_EMAIL
msg["To"] = TO_EMAIL
msg["Subject"] = SUBJECT
body = "Attached is the weekly Nessus security report in PDF format."
msg.attach(MIMEText(body, "plain"))
with open(PDF_REPORT, "rb") as attachment:
part = MIMEBase("application", "octet-stream")
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header("Content-Disposition", f"attachment; filename={os.path.basename(PDF_REPORT)}")
msg.attach(part)
server = smtplib.SMTP(SMTP_SERVER, SMTP_PORT)
server.starttls()
server.login(SMTP_USER, SMTP_PASS)
server.sendmail(FROM_EMAIL, TO_EMAIL, msg.as_string())
server.quit()
print("π© Weekly Nessus PDF Report Sent Successfully!")
# Run the script
logs = get_weekly_logs()
generate_pdf(logs)
send_email()
β Step 3: Automate Weekly PDF Report Sending
π Linux (Cron Job)
crontab -e
Add this line to run every Monday at 8 AM:
0 8 * * 1 python3 /path/to/send_weekly_nessus_report_pdf.py
π Windows (Task Scheduler)
- Create New Task β Trigger: Weekly (Monday, 8 AM)
- Action:
Start a program
- Program:
powershell.exe
- Arguments:
python "C:\path\to\send_weekly_nessus_report_pdf.py"
π‘ Final Setup Summary
β
Generates a structured PDF Nessus report π
β
Emails the report as an attachment every Monday π©
β
Keeps security reports well-documented π
Would you like to also upload this PDF to a cloud storage (Google Drive, AWS S3)? βοΈπ
πΉ Upload Nessus PDF Report to Cloud Storage βοΈπ
Now, weβll upload the weekly Nessus PDF report to Google Drive or AWS S3 for easy access and backups.
β Step 1: Choose a Cloud Storage Provider
We can upload the report to:
1οΈβ£ Google Drive (Requires Google API)
2οΈβ£ AWS S3 (Requires AWS CLI or Boto3)
β Option 1: Upload to Google Drive
Step 1.1: Install Google Drive API Library
pip install google-auth google-auth-oauthlib google-auth-httplib2 googleapiclient
Step 1.2: Get Google API Credentials
- Go to Google Cloud Console.
- Enable the Google Drive API.
- Create OAuth 2.0 credentials β Download
credentials.json
. - Place
credentials.json
in your script folder.
Step 1.3: Modify Python Script
Add this function to upload the PDF report to Google Drive:
from googleapiclient.discovery import build
from googleapiclient.http import MediaFileUpload
from google.oauth2 import service_account
# Google Drive Setup
SCOPES = ["https://www.googleapis.com/auth/drive.file"]
SERVICE_ACCOUNT_FILE = "credentials.json" # Your downloaded credentials file
def upload_to_drive():
creds = service_account.Credentials.from_service_account_file(SERVICE_ACCOUNT_FILE, scopes=SCOPES)
drive_service = build("drive", "v3", credentials=creds)
file_metadata = {"name": "Nessus_Weekly_Report.pdf", "mimeType": "application/pdf"}
media = MediaFileUpload(PDF_REPORT, mimetype="application/pdf")
file = drive_service.files().create(body=file_metadata, media_body=media, fields="id").execute()
print(f"β
Report Uploaded to Google Drive: https://drive.google.com/file/d/{file['id']}")
Step 1.4: Automate Upload After Generating Report
Call upload_to_drive()
after generating the PDF:
generate_pdf(logs)
send_email()
upload_to_drive()
β Option 2: Upload to AWS S3
Step 2.1: Install AWS SDK for Python
pip install boto3
Step 2.2: Configure AWS Credentials
aws configure
Enter your AWS Access Key, Secret Key, and Region.
Step 2.3: Modify Python Script
Add this function to upload the PDF report to AWS S3:
import boto3
# AWS S3 Configuration
S3_BUCKET = "your-s3-bucket-name"
S3_FILE_NAME = "Nessus_Weekly_Report.pdf"
def upload_to_s3():
s3 = boto3.client("s3")
s3.upload_file(PDF_REPORT, S3_BUCKET, S3_FILE_NAME)
print(f"β
Report Uploaded to S3: https://{S3_BUCKET}.s3.amazonaws.com/{S3_FILE_NAME}")
Step 2.4: Automate Upload After Generating Report
Call upload_to_s3()
after generating the PDF:
generate_pdf(logs)
send_email()
upload_to_s3()
β Final Setup Summary
β
Uploads Nessus report to Google Drive or AWS S3 βοΈ
β
Automates the process every week π
β
Ensures reports are always backed up π
Would you like to set up a Telegram bot to notify you when the report is uploaded? π’π
πΉ Get Telegram Notification When Nessus Report is Uploaded π’π
Now, weβll set up a Telegram bot to notify you when the Nessus report is uploaded to Google Drive or AWS S3.
β Step 1: Create a Telegram Bot
1οΈβ£ Open Telegram and search for BotFather.
2οΈβ£ Send /newbot
and follow the instructions.
3οΈβ£ Copy the Bot Token that BotFather provides.
4οΈβ£ Get your Chat ID by sending the bot a message and using this URL:
https://api.telegram.org/bot<Your-Bot-Token>/getUpdates
5οΈβ£ Note the chat_id from the response.
β Step 2: Install Requests Library
pip install requests
β Step 3: Modify Python Script to Send Telegram Notifications
Add this function to send a message when the report is uploaded:
import requests
# Telegram Bot Configuration
TELEGRAM_BOT_TOKEN = "YOUR_TELEGRAM_BOT_TOKEN"
TELEGRAM_CHAT_ID = "YOUR_CHAT_ID"
def send_telegram_message(message):
url = f"https://api.telegram.org/bot{TELEGRAM_BOT_TOKEN}/sendMessage"
payload = {"chat_id": TELEGRAM_CHAT_ID, "text": message}
requests.post(url, data=payload)
β Step 4: Integrate with Google Drive & AWS S3 Uploads
π If using Google Drive
Modify upload_to_drive()
function:
def upload_to_drive():
creds = service_account.Credentials.from_service_account_file(SERVICE_ACCOUNT_FILE, scopes=SCOPES)
drive_service = build("drive", "v3", credentials=creds)
file_metadata = {"name": "Nessus_Weekly_Report.pdf", "mimeType": "application/pdf"}
media = MediaFileUpload(PDF_REPORT, mimetype="application/pdf")
file = drive_service.files().create(body=file_metadata, media_body=media, fields="id").execute()
drive_link = f"https://drive.google.com/file/d/{file['id']}"
print(f"β
Report Uploaded to Google Drive: {drive_link}")
send_telegram_message(f"π’ Nessus Report Uploaded to Google Drive! π\nπ {drive_link}")
π If using AWS S3
Modify upload_to_s3()
function:
def upload_to_s3():
s3 = boto3.client("s3")
s3.upload_file(PDF_REPORT, S3_BUCKET, S3_FILE_NAME)
s3_link = f"https://{S3_BUCKET}.s3.amazonaws.com/{S3_FILE_NAME}"
print(f"β
Report Uploaded to S3: {s3_link}")
send_telegram_message(f"π’ Nessus Report Uploaded to AWS S3! π\nπ {s3_link}")
β Step 5: Automate Notification After Upload
Modify the main script:
generate_pdf(logs)
send_email()
upload_to_drive() # or upload_to_s3()
π― Final Setup Summary
β
Uploads Nessus report to Google Drive or AWS S3 βοΈ
β
Sends a Telegram notification with the report link π’
β
Automates everything weekly π