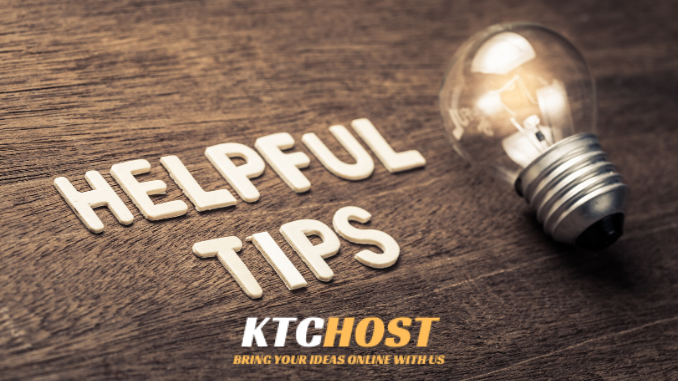
To send Slack notifications from your Jenkins pipeline based on the server load, you can integrate Slack notifications into your Jenkins job by using the Slack Notification Plugin. Here’s how you can set this up step-by-step:
Step 1: Install the Slack Notification Plugin
- Go to Jenkins Dashboard.
- Navigate to Manage Jenkins > Manage Plugins.
- Go to the Available tab and search for Slack Notification Plugin.
- Install the plugin and restart Jenkins.
Step 2: Configure Slack Integration in Jenkins
- Go to Manage Jenkins > Configure System.
- Scroll down to Slack.
- Enter the Team Domain and Integration Token from your Slack workspace:
- In Slack, go to Apps > Manage Apps > Incoming Webhooks.
- Click Add to Slack and select a channel where you want the notifications to appear.
- Copy the Webhook URL (this will be used as the Integration Token).
- Save the configuration.
Step 3: Add Slack Notification in the Jenkinsfile
Now that the Slack plugin is installed and configured, you can use it to send messages from your pipeline.
Here’s an updated version of your Jenkinsfile with Slack notifications integrated:
Example Jenkinsfile with Slack Alert on High Server Load
pipeline {
agent any
environment {
SLACK_CHANNEL = '#your-channel' // Define the Slack channel to send alerts to
SLACK_WEBHOOK_URL = 'https://hooks.slack.com/services/your/slack/webhook' // Replace with your Slack webhook URL
}
stages {
stage('Monitor Server Load') {
steps {
script {
// Using uptime command to get the load averages
def uptimeOutput = sh(script: "uptime", returnStdout: true).trim()
echo "Uptime Output: ${uptimeOutput}"
// Using top command to get the system load (1-minute load average)
def loadAverage = sh(script: "top -bn1 | grep load | awk '{printf \"%.2f\", $(NF-2)}'", returnStdout: true).trim()
echo "Current load average (1 min): ${loadAverage}"
// Check if load average is greater than a threshold (e.g., 2.0)
if (loadAverage.toFloat() > 2.0) {
echo "Warning: High server load detected!"
// Sending Slack notification about high server load
slackSend (channel: SLACK_CHANNEL,
message: "Warning: High server load detected! Current load: ${loadAverage}",
color: 'danger') // Red color for high alerts
} else {
echo "Server load is within normal range."
}
}
}
}
}
post {
success {
echo 'Server load monitoring completed successfully.'
}
failure {
echo 'Server load monitoring failed.'
}
}
}
Explanation:
- Slack Configuration in
environment
: We defineSLACK_CHANNEL
andSLACK_WEBHOOK_URL
to store Slack-related configurations. - Slack Notification (
slackSend
):- The
slackSend
step is used to send a message to Slack. channel
: The Slack channel where the alert will be sent.message
: The message that will appear in Slack. In this case, it includes the current load average.color
: You can specify the color of the message (e.g., red for high severity).
- The
Step 4: Running the Pipeline
- When the server load exceeds the threshold (e.g., 2.0 in the example), a Slack message will be sent to the defined Slack channel, notifying you of the high load.
- If the load is below the threshold, no Slack notification will be sent.
Additional Configurations:
- Additional Alerts: You can add more Slack notifications for different stages of the pipeline (e.g., success or failure).
- Customizing the Message: Customize the content of the message, such as adding more details like the server name, disk usage, or specific actions taken.
By using this setup, you can integrate real-time alerts into your Jenkins pipeline and keep your team informed about critical server metrics like load.
Would you like more guidance on configuring other types of alerts or expanding this pipeline further? if you would like your Infrastructure or application maintained by us then kindly send us email at info@ktchost.com