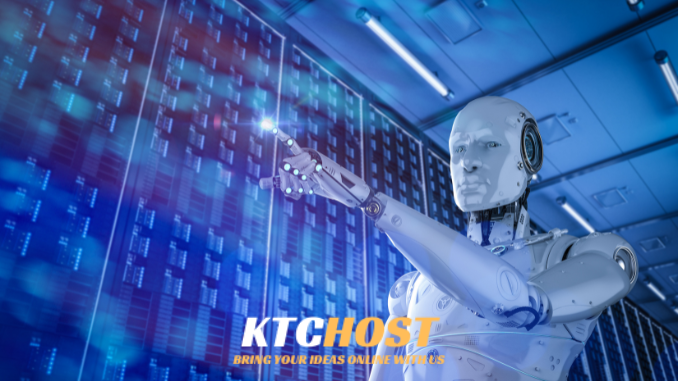
To create a Jenkins pipeline that checks the disk space and clears it if it reaches 90%, you can use shell commands inside a Jenkinsfile. You can run df
to check the disk usage and rm
or other cleanup commands to free up space. Here’s an example where we are removing the files from the /tmp directory when disk space reached 90%.
pipeline {
agent any
stages {
stage('Check Disk Space') {
steps {
script {
// Get the disk usage percentage for the root filesystem
def diskUsage = sh(script: "df / | grep / | awk '{ print \$5 }' | sed 's/%//g'", returnStdout: true).trim()
echo "Current disk usage: ${diskUsage}%"
// Check if disk usage is greater than or equal to 90%
if (diskUsage.toInteger() >= 90) {
echo "Disk usage is above 90%, performing cleanup..."
// You can perform actions here to clean up disk space, e.g., remove temporary files or old logs
sh 'sudo rm -rf /tmp/*' // Example: Remove files from /tmp directory (be cautious with this in production)
echo "Disk cleanup completed."
} else {
echo "Disk usage is below 90%, no action needed."
}
}
}
}
}
post {
success {
echo 'Disk check and cleanup pipeline succeeded.'
}
failure {
echo 'Disk check or cleanup failed.'
}
}
}
Explanation:
df /
: This command checks disk usage for the root filesystem (/
).awk '{ print $5 }'
: Extracts the percentage of disk usage.sed 's/%//g'
: Removes the percentage sign for easy comparison.diskUsage.toInteger()
: Converts the disk usage value from string to integer for comparison.- Cleanup Logic: If disk usage is over 90%, it triggers a cleanup operation (in this case, removing files from the
/tmp
directory, but you should adjust this based on your needs).
Additional Notes:
- Caution: Be careful when using commands like
rm -rf
, especially in production environments. You may want to target specific directories or use more specific cleanup methods like removing log files older than a certain number of days. - Permissions: The pipeline uses
sudo
for clearing disk space, so the Jenkins agent must have sufficient permissions to execute these commands.
This example provides a simple yet effective way to monitor disk space and automate cleanup tasks as part of a Jenkins CI/CD pipeline.
Would you like more details on disk cleanup strategies or help with adjusting the pipeline for specific needs? if you would like to outsource the Infrastructure management or Application support then kindly contact us at info@ktchost.com.